The 2016 Spring update for both Online and On-Premises, introduced the ability to capture Feedback on different entities in your CRM deployment. In this video we demonstrate what the Feedback entity is used for, and walk you through setup and configuration.
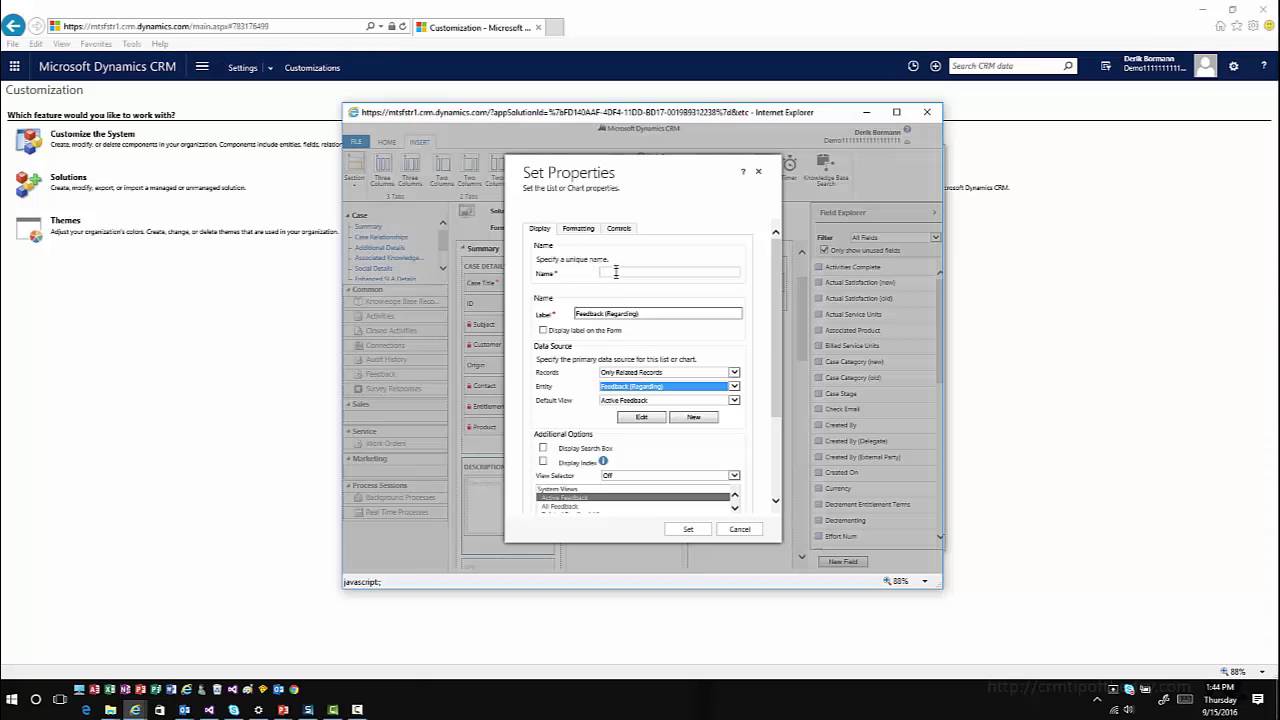
Give us your feedback, all of it: good, bad, and ugly, I’m sure we can take it. Suggest new topics either in comments or by sending your ideas to jar@crmtipoftheday.com.
Don’t forget to subscribe to http://youtube.com/crmtipoftheday.