In this video we look at how to create a PowerApp, and connect to the Dynamics 365 instance. We talk about some of the basic commands available and how to work with them.
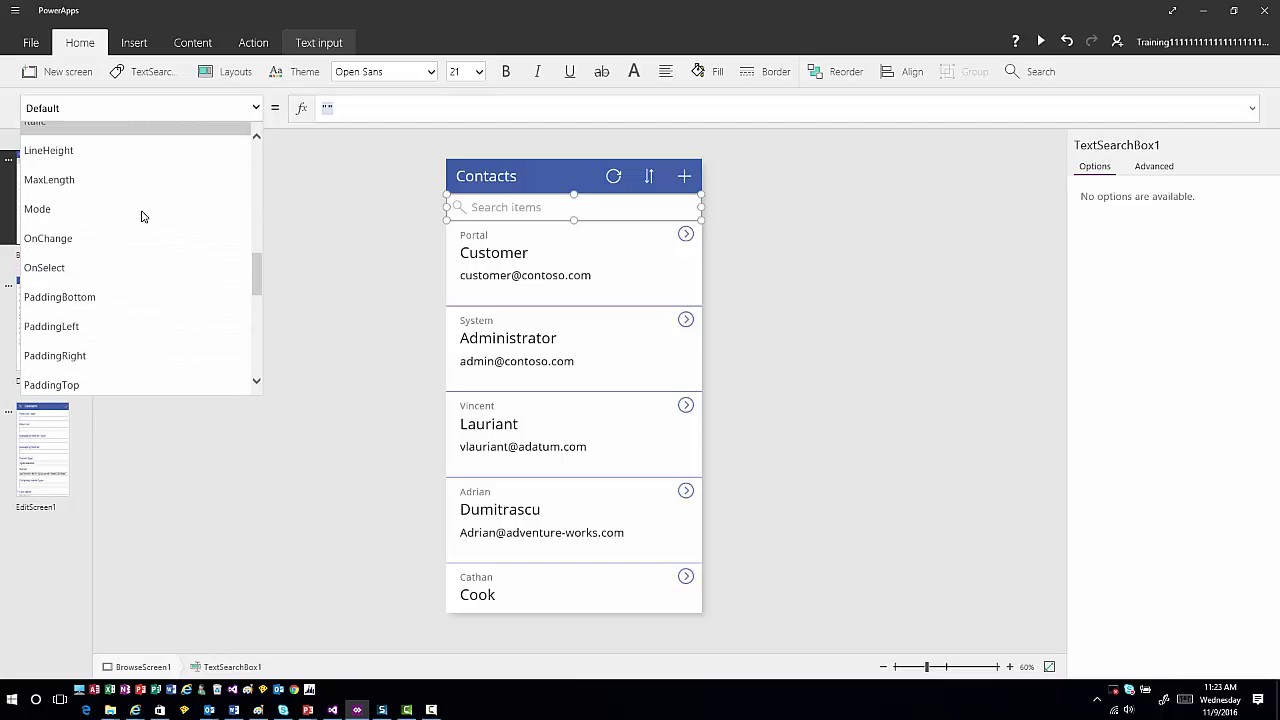
Give us your feedback, all of it: good, bad, and ugly, I’m sure we can take it. Suggest new topics either in comments or by sending your ideas to jar@crmtipoftheday.com.
Don’t forget to subscribe to http://youtube.com/crmtipoftheday.